Facebook, with its billions of active users, is a goldmine of valuable social data. While accessing publicly available posts is relatively straightforward, scraping Facebook comments presents a unique set of challenges and opportunities.
Understanding the sentiments, opinions, and trends expressed in Facebook comments can provide businesses and researchers with crucial insights into customer behavior, brand perception, and public discourse. However, navigating Facebook’s terms of service and evolving API restrictions requires careful consideration and a strategic approach.
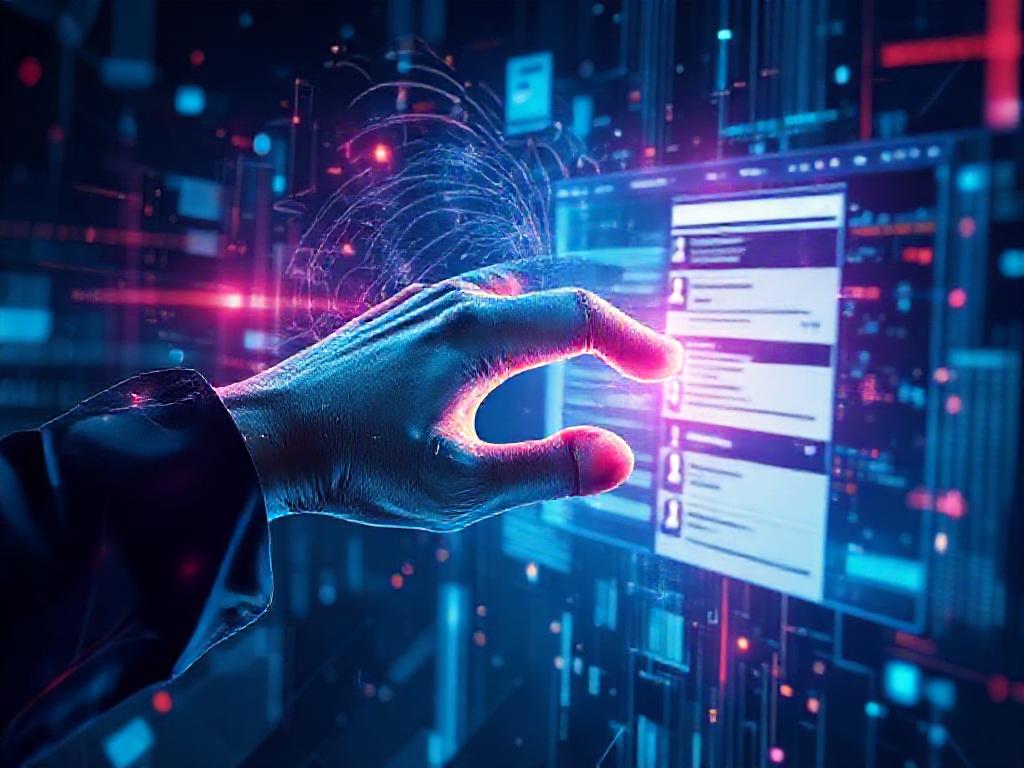
Why Scrape Facebook Comments?
Extracting Facebook comment data can unlock a wealth of information for various purposes:
- Market Research: Gauge customer satisfaction, identify trending topics, and analyze public opinion towards products, services, or campaigns.
- Competitive Analysis: Monitor competitor brand mentions, track customer feedback on their offerings, and identify areas for improvement.
- Sentiment Analysis: Understand the overall sentiment expressed towards your brand, products, or industry, allowing you to tailor your strategies accordingly.
- Social Listening: Track conversations around specific keywords, hashtags, or events to gain real-time insights into public perception and emerging trends.
- Content Curation: Discover valuable user-generated content, identify popular themes, and create engaging content that resonates with your target audience.
Challenges of Scraping Facebook Comments
Scraping Facebook comments presents several hurdles:
- Facebook’s Anti-Scraping Measures: Facebook actively combats automated data extraction, implementing measures like IP blocking, CAPTCHAs, and rate limiting to prevent excessive scraping.
- Dynamic Content Loading: Facebook comments are often loaded dynamically using JavaScript, requiring more sophisticated scraping techniques to capture all data.
- Complex Data Structures: Facebook comment sections have intricate HTML structures, making it challenging to extract specific data points like user names, timestamps, and comment text reliably.
- Evolving API Landscape: Facebook frequently updates its API, which may impact existing scraping methods and require adjustments to your code.
Ethical Considerations
It’s crucial to approach Facebook comment scraping ethically:
- Respect Facebook’s Terms of Service: Always adhere to Facebook’s terms of service and avoid activities that violate their policies.
- Obtain User Consent (When Possible): When scraping personal data, consider obtaining explicit consent from users, especially if you intend to use their information for commercial purposes.
- Use Data Responsibly: Protect user privacy and handle scraped data responsibly. Avoid sharing sensitive information without proper authorization.
Techniques for Scraping Facebook Comments
Here are some techniques commonly used for scraping Facebook comments:
- Web Scraping Libraries: Utilize Python libraries like Beautiful Soup and Scrapy to parse HTML content and extract relevant data.
- Selenium: Employ web automation tools like Selenium to interact with Facebook pages as if a human user were browsing, allowing you to scrape dynamic content.
- Facebook Graph API: Leverage Facebook’s official API to access public comment data. However, be aware of API limitations and rate restrictions.
- Browser Extensions: Explore browser extensions specifically designed for scraping Facebook comments. These can simplify the process but may have limitations in terms of functionality and data extraction.
Example: Scraping Comments Using Beautiful Soup and Requests
This example demonstrates a basic approach to scraping comments using Python’s requests
and Beautiful Soup
libraries.
import requests
from bs4 import BeautifulSoup
url = 'https://www.facebook.com/examplepage/post_id'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
comments = []
for comment_element in soup.find_all('div', class_='comment'):
user_name = comment_element.find('a', class_='user-name').text.strip()
comment_text = comment_element.find('div', class_='comment-text').text.strip()
comments.append({'user': username, 'text': comment_text})
print(comments)
Note: This code is for illustrative purposes only and may require adjustments based on Facebook’s evolving HTML structure.
Key Takeaways
- Scraping Facebook comments can provide valuable insights for businesses and researchers.
- Ethical considerations and respect for Facebook’s terms of service are paramount.
- Web scraping techniques, including libraries like Beautiful Soup and Selenium, can be used to extract data.
- Facebook’s dynamic content loading and anti-scraping measures require careful consideration and adaptation.
Frequently Asked Questions (FAQs)
Scraping Facebook comments can be legal if conducted ethically and in compliance with Facebook’s terms of service. Always review their policies to ensure your activities are permissible.
No, scraping private comments violates Facebook’s terms of service and is considered unethical.
Popular tools include Beautiful Soup, Scrapy, Selenium, and specialized browser extensions designed for Facebook scraping.
Facebook enforces rate limits to prevent server overload. Adhering to these limits is essential to avoid being blocked from accessing the site.