Playwright is a powerful and flexible open-source node.js library developed by Microsoft for automating web browsers. It supports Chromium, Firefox, and WebKit browsers, enabling developers to perform end-to-end testing, web scraping, and automation tasks with ease. With its ability to simulate multiple scenarios, handle complex interactions, and maintain stability across different browsers, Playwright has become a popular choice for professionals and businesses in the web scraping community.
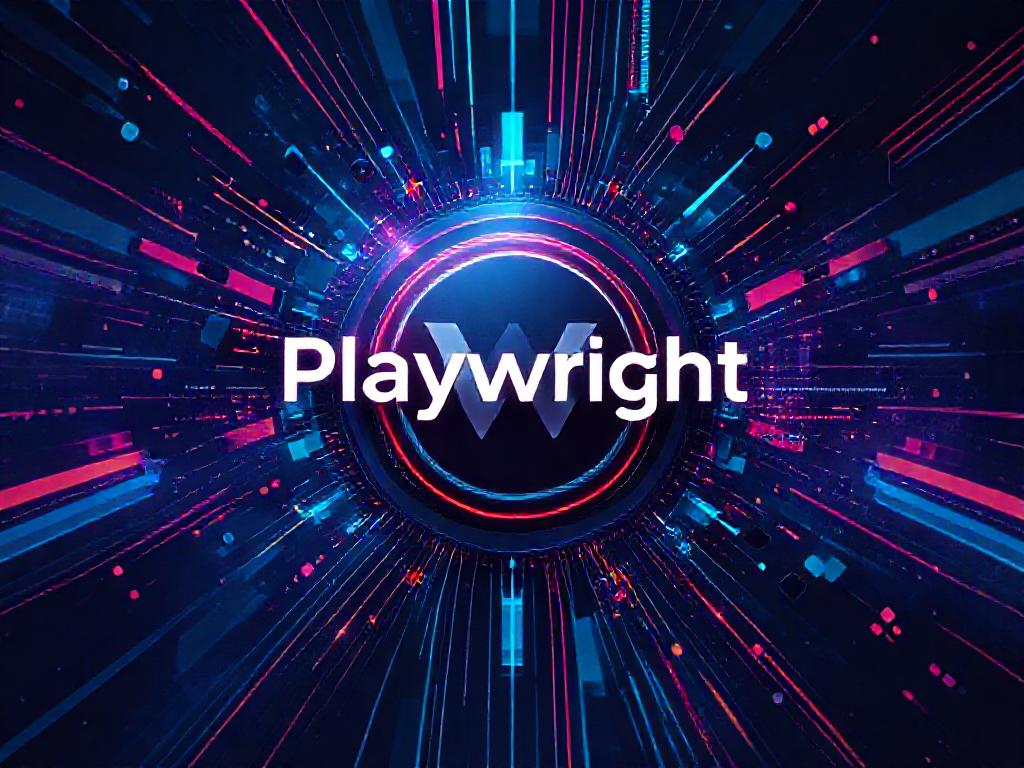
Introduction to Playwright
Playwright is an automation library that controls headless and full (non-headless) web browsers, enabling developers to automate tasks such as clicking buttons, filling out forms, and navigating web pages. It builds on the foundations of its predecessor, Puppeteer, by offering additional features and capabilities, including multi-browser support, faster performance, and more extensive automation options.
Playwright allows you to:
- Perform end-to-end testing: Test your web applications across different browsers, ensuring compatibility and stability.
- Automate web navigation: Simulate user interactions and automate repetitive tasks for web scraping and data extraction.
- Generate screenshots and PDFs: Capture web pages as images or PDF files for documentation and reporting purposes.
Key Features and Benefits
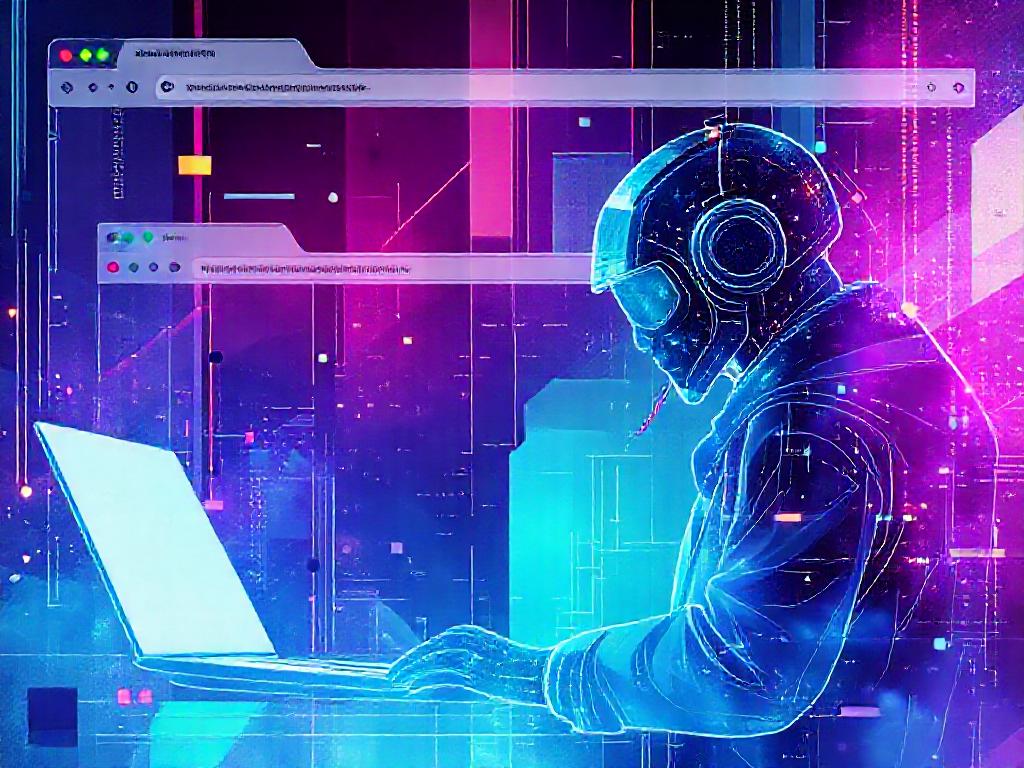
- Cross-browser compatibility: Playwright supports Chromium, Firefox, and WebKit browsers, ensuring consistent automation across various platforms.
- Fast and reliable: Playwright offers faster performance and higher stability compared to other automation libraries, reducing the chances of failed executions.
- Interaction automation: Playwright can automate complex interactions such as mouse movements, keyboard input, and touch gestures.
- Network interception: Inspect and modify network requests and responses for debugging, testing, or data manipulation purposes.
- Element selection: Playwright provides multiple methods for selecting and interacting with elements, including CSS selectors, XPath, and text matching.
- Browser contexts: Manage multiple browser contexts to isolate cookies, local storage, and other data for better automation control.
- Device emulation: Simulate various devices, screen sizes, and orientations for testing and automating responsive web applications.
Getting Started with Playwright
Before diving into the world of Playwright, ensure that you have the following prerequisites:
- Node.js (version 12.0.0 or later)
- npm (Node Package Manager)
You can install Playwright by running the following command in your terminal or command prompt:
npm i playwright
Playwright API and Usage
Playwright provides a simple and intuitive API for automating web browsers. Here’s a basic example of launching a browser and navigating to a webpage:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await browser.close();
})();
Playwright also supports other browsers, such as Firefox and WebKit, through their respective objects:
const { firefox } = require('playwright');
const { webkit } = require('playwright');
Web Scraping with Playwright
Playwright can be an effective tool for web scraping due to its automation capabilities and robust feature set. To extract data from a webpage, you can use the following steps:
- Launch a browser and open the web page.
- Select elements containing the desired data.
- Extract the data from the selected elements.
Here’s a basic example of web scraping using Playwright:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Select all table rows
const rows = await page.$$eval('table tbody tr', (elements) => {
return elements.map((row) => {
// Extract text from each cell
const cells = row.querySelectorAll('td');
return Array.from(cells).map((cell) => cell.innerText);
});
});
console.log(rows);
await browser.close();
})();
Best Practices and Tips
- Avoid excessive requests: Respect the website’s rate limits and use delays between requests to prevent overwhelming the server.
- Handle errors and timeouts: Implement error handling and timeouts to ensure that your web scrapers can adapt to unpredictable situations.
- Rotate IP addresses: Use rotating proxies or residential IPs to avoid detection and maintain the longevity of your web scraping projects.
- Respect robots.txt: Adhere to the website’s robots.txt file to avoid violating their terms of service.
- Mimic user behavior: Simulate human-like interactions such as random delays, mouse movements, and scrolling to reduce the chance of being blocked.
Comparing Playwright with Other Tools
When comparing Playwright with other web scraping tools, consider the following factors:
- Cross-browser compatibility: Playwright supports multiple browsers, while tools like Puppeteer and Selenium primarily focus on Chromium-based browsers.
- Performance: Playwright offers faster performance compared to Puppeteer and Selenium, reducing the overall execution time of your scripts.
- Ease of use: Playwright has a simple and intuitive API, making it easier for developers to get started with automation and web scraping tasks.
Playwright Use Cases
Playwright can be applied in various scenarios, such as:
- Web scraping and data extraction
- Automated testing and quality assurance
- Generating screenshots and PDFs for documentation
- Simulating and testing responsive web applications
- Automating repetitive tasks for productivity enhancement
Playwright Ecosystem and Resources
To learn more about Playwright and stay updated with its latest features, consider the following resources:
- Playwright documentation
- Microsoft Playwright GitHub repository
- Playwright community on GitHub Discussions
Frequently Asked Questions
Yes, Playwright is completely free and open-source. Developed by Microsoft, it allows developers to utilize and contribute to its codebase without incurring any licensing fees, promoting a collaborative environment for software development.
Absolutely! Playwright is an exceptional tool for web scraping due to its powerful automation capabilities and support for handling dynamic content. It enables users to extract data from websites efficiently, making it suitable for various applications, from data collection to competitive analysis.
Playwright supports a variety of browsers, including Chromium, Firefox, and WebKit (Safari). This multi-browser support ensures cross-browser compatibility and allows developers to automate tasks across different environments seamlessly.
Playwright provides several advantages over Puppeteer, including broader multi-browser support, enhanced performance, and more extensive automation features. While Puppeteer is primarily focused on Chrome, Playwright’s ability to operate across multiple browsers makes it a more versatile choice for developers.
Error handling and timeouts in Playwright can be effectively managed using try-catch blocks and setting explicit timeouts for various actions and navigation processes. This approach allows developers to create robust scripts that can gracefully handle unexpected issues during execution.